diff options
author | Nicholas Van Doorn <vandoorn.nick@gmail.com> | 2018-11-15 13:10:01 -0800 |
---|---|---|
committer | GitHub <noreply@github.com> | 2018-11-15 13:10:01 -0800 |
commit | 158fd00543e468675c83f9ad23a4ce47a716f94f (patch) | |
tree | 9f75c16e478a1518f7f84284678564823dc04e15 /readme.md | |
parent | 3da7cd6011d084fcb72b6f696319374ec59c2cd6 (diff) | |
parent | 4e379259292be2709834ee0d75e212f80b14e1b0 (diff) |
Merge pull request #8 from brnkl/documentation
Documentation
Diffstat (limited to 'readme.md')
-rw-r--r-- | readme.md | 76 |
1 files changed, 76 insertions, 0 deletions
@@ -0,0 +1,76 @@ +MangOH Motion Service +====== +This application is designed to operate on a [MongOH Red](https://mangoh.io/mangoh-red-new) WP85 board. Using **general input output** this project uses pthreads to probe the onboard bmi160 chip intermittently in order to detect a significant motion on the board. + +## Prerequisites + +When cloning use ``git clone https://github.com/brnkl/motion-service.git``. + + +## Building +Compile the project using + +wp85: ``make wp85`` + +## Setting Variables +To change the sensitivity of impact detection you must edit the `motionMonitor/motionMonitor.c` file. +The Accelerometer measures acceleration in 3 dimensions, X, Y, and Z. These dimensions of acceleration are recorded and the magnitude of their resulting vector is calculated using ``double impactMagnitude = sqrt(x * x + y * y + z * z);``. As visualized in the image below. + +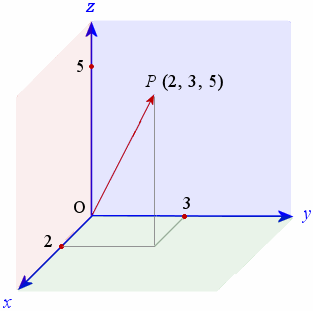 + + +`#define DEFAULT_THRESHOLD_MS2 17` determines the magnitude of the resulting vector that will trigger the application to detect a sudden impact. + +If adjusting the value of `DEFAUTL_THRESHOLD_MS2` keep in mind that gravity implies a motionless magnitude of -9.8m/s^2. + +## Bindings +App.adef +``` +... +bindings: +{ + myClientApp.myComponent.brnkl_motion -> motionService.brnkl_motion +} +... +``` +Component.cdef +``` +... +requires: +{ + api: + { + brnkl_motion.api + } +} +... +``` + +## Example +For getting the current acceleration +```C +struct Acceleration{ + double x; + double y; + double z; +}; + +struct Acceleration v = value; + +le_result_t result = brnkl_motion_getCurrentAccleration(&v->x, &v->y, &v->z); +``` +To get a list of all recent impacts. + +``` + double xAcc[N_MAX_IMPACT_VALUES], yAcc[N_MAX_IMPACT_VALUES], zAcc[N_MAX_IMPACT_VALUES]; + + uint64_t timestamps[N_MAX_IMPACT_VALUES]; + + size_t xSize = N_MAX_IMPACT_VALUES, ySize = N_MAX_IMPACT_VALUES, + zSize = N_MAX_IMPACT_VALUES, timestampsSize = N_MAX_IMPACT_VALUES; + + le_result_t r = brnkl_motion_getSuddenImpact( + xAcc, &xSize, yAcc, &ySize, zAcc, &zSize, timestamps, ×tampsSize); + +``` + |